7 things all devops practitioners need from Git
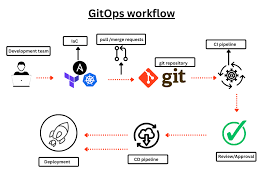
Git is a powerful tool for version control, enabling multiple developers to work together on the same codebase without stepping on each other’s toes. It’s a complex system with many features, and getting to grips with it can be daunting. Here are seven insights that I wish I had known when I started working with Git.
The Power of git log
The git log
command is much more powerful than it first appears. It can show you the history of changes in a variety of formats, which can be extremely helpful for understanding the evolution of a project.
# Show the commit history in a single line per commit
git log --oneline
# Show the commit history with graph, date, and abbreviated commits
git log --graph --date=short --pretty=format:'%h - %s (%cd)'
Branching is Cheap
Branching in Git is incredibly lightweight, which means you should use branches liberally. Every new feature, bug fix, or experiment should have its own branch. This keeps changes organized and isolated from the main codebase until they’re ready to be merged.
# Create a new branch
git branch new-feature
# Switch to the new branch
git checkout new-feature
Or do both with:
# Create and switch to the new branch
git checkout -b new-feature
git stash
is Your Friend
When you need to quickly switch context but don’t want to commit half-done work, git stash
is incredibly useful. It allows you to save your current changes away and reapply them later.
# Stash your current changes
git stash
# List all stashes
git stash list
# Apply the last stashed changes and remove it from the stash list
git stash pop
git rebase
for a Clean History
While merging is the standard way to bring a feature branch up to date with the main branch, rebasing can often result in a cleaner project history. It’s like saying, “I want my branch to look as if it was based on the latest state of the main branch.”
# Rebase your current branch on top of the main branch
git checkout feature-branch
git rebase main
Note: Rebasing rewrites history, which can be problematic for shared branches.
The .gitignore
File
The .gitignore
file is crucial for keeping your repository clean of unnecessary files. Any file patterns listed in .gitignore
will be ignored by Git.
# Ignore all .log files
*.log
# Ignore a specific file
config.env
# Ignore everything in a directory
tmp/**
git diff
Shows More Than Just Differences
git diff
can be used in various scenarios, not just to show the differences between two commits. You can use it to see changes in the working directory, changes that are staged, and even differences between branches.
# Show changes in the working directory that are not yet staged
git diff
# Show changes that are staged but not yet committed
git diff --cached
# Show differences between two branches
git diff main..feature-branch
The Reflog Can Save You
The reflog is an advanced feature that records when the tips of branches and other references were updated in the local repository. It’s a lifesaver when you’ve done something wrong and need to go back to a previous state.
# Show the reflog
git reflog
# Reset to a specific entry in the reflog
git reset --hard HEAD@{1}
Remember: The reflog is a local log, so it only contains actions you’ve taken in your repository.
Understanding these seven aspects of Git can make your development workflow much more efficient and less error-prone. Git is a robust system with a steep learning curve, but with these tips in your arsenal, you’ll be better equipped to manage your projects effectively.