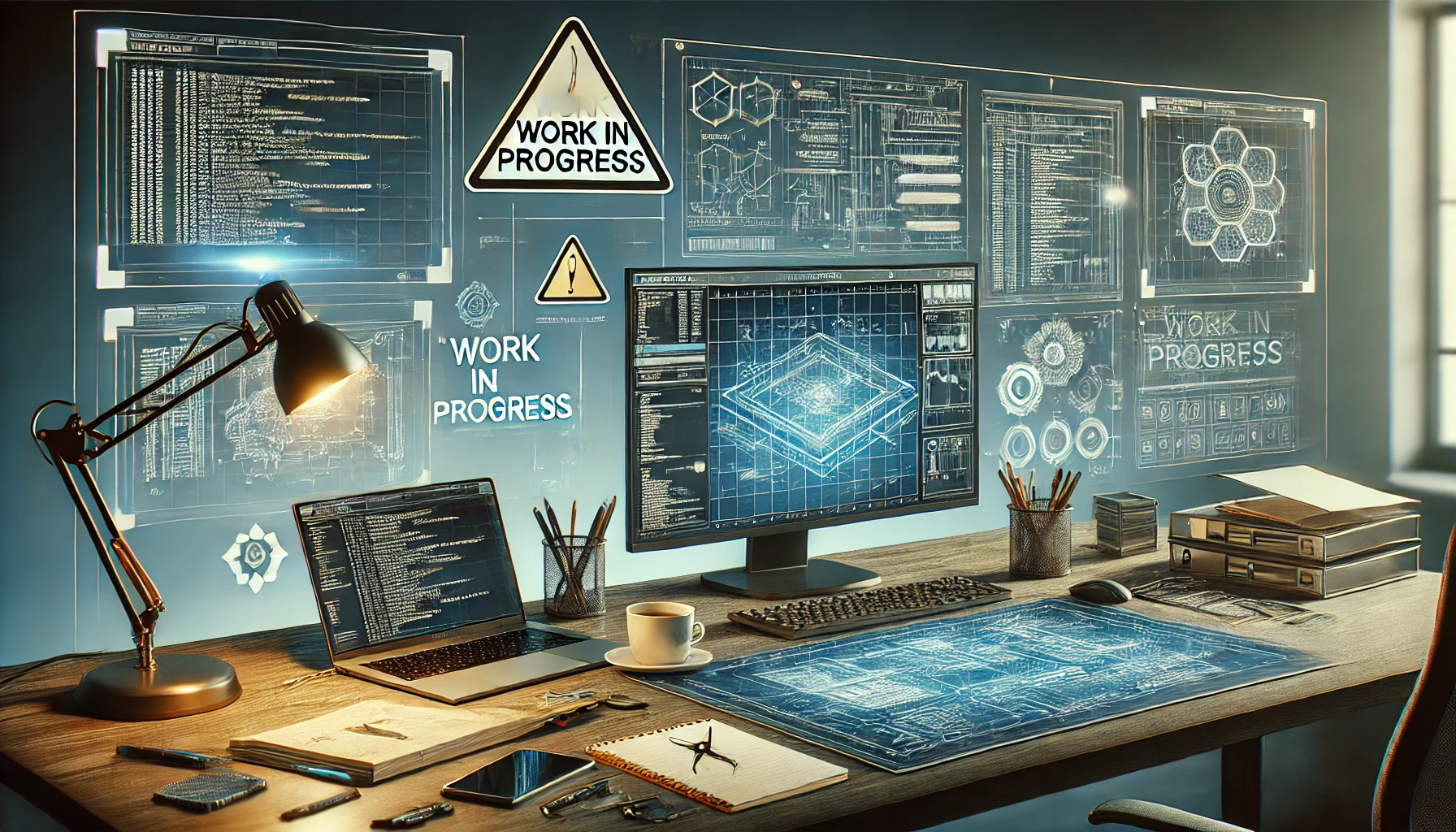
Welcome! Setting up a development environment is the first crucial step towards efficient and productive coding. In this blog post, we will walk you through the process of setting up a development environment, covering essential tools, configurations, and tips to get you started.
Why a Good Development Environment Matters
A well-configured development environment can significantly boost your productivity by providing the necessary tools and workflows to write, test, and debug code efficiently. It also helps in maintaining consistency across different projects and teams.
1. Choosing the Right Operating System
Your choice of operating system (OS) can influence your development experience. The three most common options are:
- Windows: Popular for its user-friendly interface and compatibility with various software.
- macOS: Preferred by many developers for its Unix-based system and seamless integration with Apple hardware.
- Linux: Highly customizable and open-source, making it a favorite among developers who prefer full control over their environment.
Resources:
2. Installing Essential Tools
Here are some essential tools you’ll need in your development environment:
Code Editor/IDE:
- Visual Studio Code (VS Code): Lightweight and highly customizable. Download VS Code
- JetBrains IntelliJ IDEA: Powerful IDE, especially for Java and Kotlin. Download IntelliJ IDEA
Version Control System:
- Git: Essential for source code management. Download Git or “zypper in git-core“
Package Managers:
- zypper (openSUSE): Choose from a number of maintained packages.
- Homebrew (macOS/Linux): Simplifies software installation. Install Homebrew
- Chocolatey (Windows): Easy software management on Windows. Install Chocolatey
Terminal Emulator:
- Windows Terminal: A modern terminal for Windows. Install Windows Terminal
- iTerm2: A popular terminal for macOS. Download iTerm2
3. Setting Up Git and GitHub
Git is a crucial tool for version control and collaboration. Setting up Git and connecting it to GitHub is essential.
Installing Git:
# On macOS using Homebrew
brew install git
# On openSUSE
sudo zypper in git-core
# On Windows (via Chocolatey)
choco install git
Configuring Git:
# Set your username and email
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Connecting to GitHub:
- Create a GitHub account.
- Generate an SSH key.
- Add the SSH key to your GitHub account: Adding a new SSH key.
4. Configuring Your Code Editor/IDE
Visual Studio Code (VS Code):
- Install Extensions:
- Python: For Python development.
- Prettier: Code formatter.
- ESLint: JavaScript linter.
- VS Code Marketplace
- Settings Sync: Sync your settings across multiple machines. Settings Sync
IntelliJ IDEA:
- Plugins: Install necessary plugins for your development stack.
- Themes and Keymaps: Customize the appearance and shortcuts. IntelliJ Plugins
5. Setting Up Your Development Stack
Depending on your technology stack, you will need to install additional tools and libraries.
For JavaScript/Node.js Development:
- Node.js: JavaScript runtime. Download Node.js
- npm: Node package manager, included with Node.js.
- yarn: Alternative package manager. Install Yarn
For Python Development:
- Python: Install the latest version. Download Python
- pip: Python package installer, included with Python.
- Virtualenv: Create isolated Python environments. Virtualenv Documentation
For Java Development:
- JDK: Java Development Kit. Download JDK
- Maven/Gradle: Build tools. Maven, Gradle
6. Configuring Development Environments for Web Development
Setting Up a LAMP Stack on Linux:
- Apache: Web server.
- MariaDB: Database server.
- PHP: Scripting language.
sudo zypper ref
sudo zypper in apache2
sudo zypper in mariadb mariadb-tools
sudo zypper in php libapache2-mod-php php-mysql
Setting Up a MEAN Stack:
- MongoDB: NoSQL database.
- Express.js: Web framework for Node.js.
- Angular: Front-end framework.
- Node.js: JavaScript runtime.
# Install MongoDB
brew tap mongodb/brew
brew install mongodb-community@5.0
# Install Express.js and Angular CLI
npm install -g express-generator @angular/cli
Conclusion
Setting up a robust development environment is the cornerstone of efficient software development. By following the steps outlined in this post, you’ll have a well-configured environment tailored to your needs, ready to tackle any project.
Additional Resources:
Stay tuned for more tutorials and guides to enhance your development experience. Happy coding!